Deploying EC2 Instances with Terraform and Security Groups in AWS 2025
Introduction
Terraform is an open-source Infrastructure as Code (IaC) tool that uses a declarative configuration language to let users define and provision cloud infrastructure. This post explains how to set up security groups, deploy EC2 instances in AWS, and specify output values for obtaining instance information.
Objectives:
- Deploy EC2 instances in AWS.
- Set up security groups to control both outgoing and incoming traffic.
- Define output values to retrieve instance information effectively.
- Automate the deployment of infrastructure to improve management and scalability.
Prerequisites:
Before proceeding, ensure you have:
- An AWS account
- Terraform installed on your system
- AWS CLI configured with appropriate credentials
Note:-
Change the ami_id variable to match your required AMI.
Modify the security group rules as per your requirements.
ALSO READ:
- How to Launch an EC2 Instance: Create a Key Pair and Configure Security Group.
- How to install Linux Server Monitoring tool Nagios XI and NCPA
- Step-by-Step Git Workflow: Managing a Project with Git and GitHub 2024
Configuration Breakdown
- Defining Variables
We define variables to parameterize our infrastructure, making it reusable and scalable.
variable "ami_id" { type = string default = "ami-09c813fb71547fc4f" description = "This is AMI ID for RHEL9" } variable "instance_name_loop" { type = list(any) default = ["mysql", "frontend", "backend"] description = "List of instance names" } variable "environment" { type = string default = "dev" description = "Environment variable" } variable "instance_type" { type = string default = "t2.micro" description = "EC2 instance type" }
- Creating an EC2 Instance
The aws_instance resource defines three EC2 instances using the count parameter. Each instance is assigned a unique name from the instance_name_loop variable.
resource "aws_instance" "foo" { count = 3 ami = var.ami_id vpc_security_group_ids = [aws_security_group.allow_tl.id] instance_type = var.environment == "prod" ? "t3.micro" : "t2.micro" tags = { Name = var.instance_name_loop[count.index] } }
- Creating a Security Group
We define an AWS security group allowing SSH traffic and enabling outbound access.
resource "aws_security_group" "allow_tl" { name = "allow_tl" description = "Allow SSH inbound traffic and all outbound traffic" ingress { from_port = 22 to_port = 22 protocol = "tcp" cidr_blocks = ["0.0.0.0/0"] } egress { from_port = 0 to_port = 0 protocol = "-1" cidr_blocks = ["0.0.0.0/0"] } tags = var.sg_tags }
- Defining Output Values
We use output variables to retrieve helpful information, such as instance IP addresses and security group IDs.
output "security_group_id" { description = "Security group ID" value = aws_security_group.allow_tl.id } output "instance_ip_mapping" { description = "Instance IPs" value = { for instance, name in var.instance_name_loop : name => { private_ip = aws_instance.foo[instance].private_ip public_ip = aws_instance.foo[instance].public_ip } } }
Deploying the Infrastructure
- Initialize : Prepares the working directory.
terraform init
- Validate Configuration: Checks for syntax errors.
terraform validate
- Plan Deployment: Preview the changes before applying.
terraform plan
- Apply Configuration: Deploys the infrastructure.
terraform apply -auto-approve
- Verify Output: Displays instance details.
terraform output
Example Screenshots:
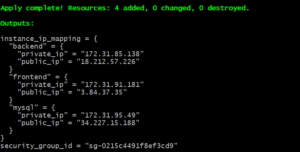
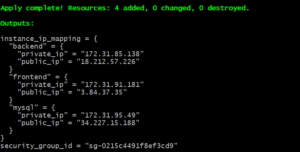